Graduate Certificate in Full Stack Development with MVC & Blazor UI
Arrange the following work environment for work-from-home training.
Why Learn Full Stack Development with MVC & Blazor UI?
Object-Oriented Programming
Windows Forms programming ADO.Net
LINQ, WCF and Web Services
Variables, Operators, and Flow Control
Advanced Topics – Error handling
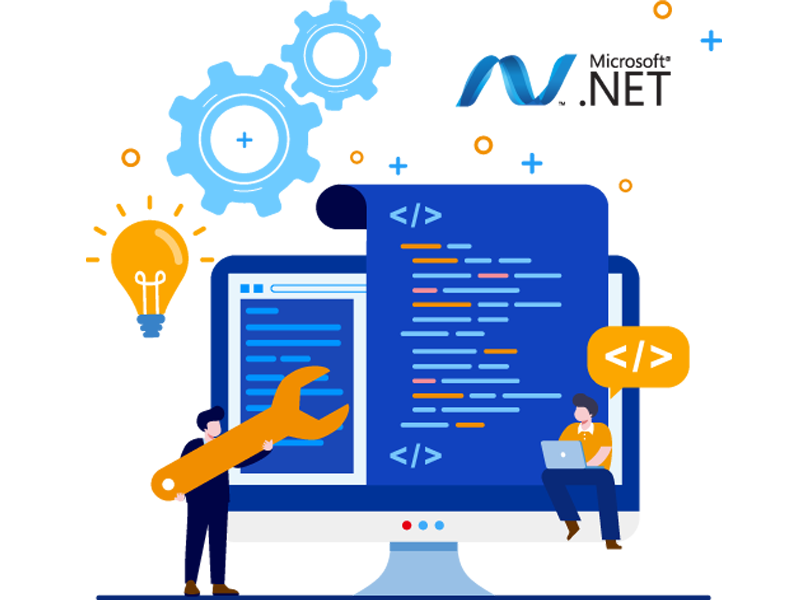
+
0
$
Student
+
0
$
professional
75%
Our Services
Unlocking Success ThroughFull stack Development
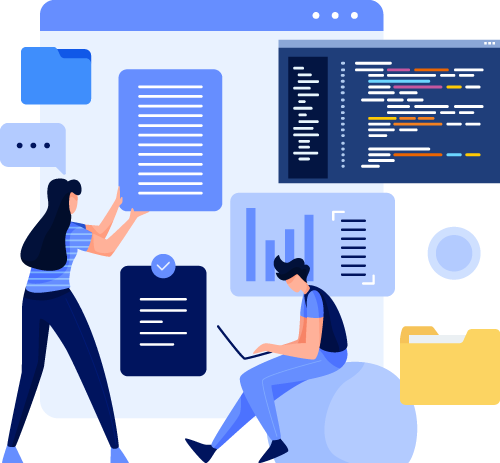
Introduction to Blazor
Blazor empowers developers to construct any type of application, ranging from static websites (akin to ASP.NET MVC) to highly interactive Single Page Applications, as well as desktop and mobile applications. Blazor truly has it all! In this overview, we’ll take a high-level look at the features Blazor offers for building the websites of the future!
- Short introduction to Razor syntax
- Blazor Components replacing MVC Controllers and Views.
- Converting a static web site with Blazor.
- Adding Streamed Rendering to improve user experience.
- Using Blazor to add interactivity to part of the web site.
- Introducing Web Assembly with .NET and Blazor.
- Introducing the different application models.
- Blazor Features Overview.
- Using Visual Studio Templates to Get Started.
- LAB: Getting started with Blazor.
Blazor Components
In modern web development, we construct applications from components, which, in turn, are often composed of smaller components. A Blazor component is a self-contained segment of the user interface, designed with a single responsibility. Blazor components, crafted from Razor and C#, offer ease of understanding, debugging, and maintenance. Naturally, these components can be reused across different pages, enhancing development efficiency.
- What is a Blazor Component?
- Building a Simple Blazor Component
- Component Parameters.
- Conditional Rendering and Child Content.
- Separating the View and View-Model.
- Styling Components
- LAB: Using components for building a simple website
Blazor Data Binding
Modern web applications frequently adopt the Model-View-ViewModel (MVVM) approach, underscoring the critical role of data binding. Blazor aligns seamlessly with this trend! In the upcoming chapter, we will delve into the diverse methods of data binding that Blazor offers.
- A Quick Look at Razor
- One Way Data Binding
- Event Handling and Data Binding
- Two Way Data Binding
- Reporting Changes with State Has Changed
- Component Data Binding
- Understanding Event Call back
- LAB: Using Data Binding
Understanding Blazor Component Lifecycle Hooks
Blazor components are created, undergo changes, and are eventually removed. In this section, we will explore the points within a component’s lifecycle where you can intercept and interact with these stages.
- Understanding Lifecycle Hooks
- Implementing the right LifeCycle Hook(s)
- Limiting unnecessary Rerendering of components with Should Render
- LAB: Implementing Life Cycle Hooks
Services and DependencyInjection.
Dependency Inversion is a foundational principle of sound Object-Oriented design, with Dependency Injection serving as its crucial facilitator. In this chapter, we will delve into both dependency inversion and injection, exploring their essential roles in Blazor architecture. We will demonstrate these concepts by developing a service that abstracts the processes of data retrieval and storage. Such services are pivotal in enabling components to operate efficiently both on the server and in WebAssembly (WASM).
- Understanding Dependency Inversion & Injection
- Some Inversion of Control Containers
- Blazor and Dependency Injection
- Building Blazor Services
- LAB: Create a service to talk to the data store
Advanced Blazor Components
Blazor components are the foundational elements for building websites. But how can you create components that are truly reusable? And what considerations should be made for performance?
- Referring to Components
- Building Templated Components
- Advanced Templated Components with Piggy-Wig
- Optimized Rendering with Razor Templates
- Rendering large amounts of rows using Virtualization
- Help Blazor with change detection using @key
- LAB: Building a Templated Component
Built-in Blazor Components
What components are included with Blazor out-of-the-box? Is it possible to dynamically select components for use? Furthermore, does Blazor offer a component for displaying a data table that supports sorting and filtering? It’s worth noting that we will focus on components not discussed elsewhere.
- Using Existing Blazor Component Libraries
- Using Dynamic Component
- HeadContent and HeadOutlet
- Feedback with Error Boundaries
- Setting default focus with FocusOnNavigate
- InputFile of uploading contents
- Showing tables of data with QuickGrid
- LAB: Using QuickGrid